Role of Aggregators and Subcommittees
A voluntary role to reduce bandwidth requirements on the network
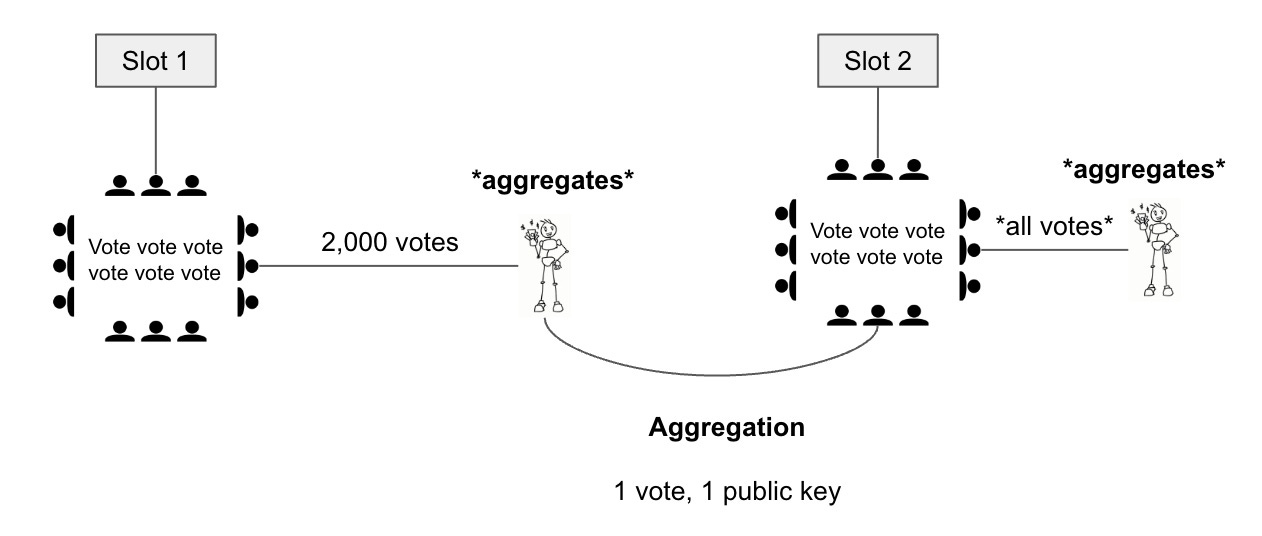
All attestations are recorded in the beacon blockchain. This serves to maintain a copy of the protocol transcript to allow anyone to verify the weight of this blockchain fork. It also guarantees the attestations are available for Validators in future slots when they apply the fork-choice rule.
Interestingly, there a single restriction placed on a block proposer who is including attestations in a beacon block:
Limited attestations. A beacon block only allocates ~32KB for attestation data and it can only store up to ~128 attestations.
A restriction of 32 KB may not seem daunting at first, but let’s consider the total attestation data that is produced within a single slot:
The average committee size for a slot is around 15k Validators who will produce 15k unique attestations,
A single attestation is approximately 250 bytes and for 15k attestations it is roughly 3.75MB.
This is an order of magnitude difference.
It is simply not possible to store every unique attestation in the next beacon block as 3.75MB > 32 KB. It is important too as a ~4MB every 12 seconds — on just voting data — is needlessly wasteful and will unnecessarily blow up the blockchain’s size. The solution to reduce the data size is to aggregate all attestation data before including it in a beacon block.
There is a single component that makes aggregation viable:
Aggregation of digital signatures. BLS was chosen as the digital signature scheme as it supports non-interactive aggregation. Anyone can take a list of digital signatures for the same message and reduce it to a single digital signature. All public keys are also aggregated into a single public key.
It is up to a new special agent called aggregators to collect the digital signatures, aggregate them, and pass it onto the next slot. They are elected using a private lottery protocol and can decide whether they want to take on the role during this slot. It is voluntary, there is no rewards or on-chain acknowledgement for performing it.
An aggregator does not collect signatures for the entire committee of Validators. In fact, this brings us to a subtle detail that a slot’s committee is not a single committee, but it is made up of 64 smaller committees (subcommittees*). They are only responsible for aggregating signatures for their allocated subcommittee. This reduces the bandwidth requirements as they only expected to handle around 240 attestations (15k per slot / 64 committees).
*We use the term subcommittee for convenience, but this terminology is not used by the ETH2 researchers (yet!, hehehe).
Aggregation of BLS digital signatures
All attestations rely on the BLS digital signature scheme and it has a special ability to allow the aggregation of signatures from different public keys as long as they have all signed the same message.
There are three functions:
Signing function
σ=SIGN(M, sk)
Input → A message
M
and the signer’s secret keysk
.Output → A digital signature
σ.
Verify function
b=VERIFY(σ, P)
Input → A message
M
and the signer’s public keyP
.Output → A boolean
b
onw
hether it was a valid signature on the message.
Aggregate function
(P,
σ)=aggregate(
[(P1,σ1), …, (Pn, σn)])
Input → A list of public keys and signatures.
Output → A single public key and signature.
The attestation cast by the Validator is represented by the message M
. Within a single slot, there can be multiple competing attestations M,…,M’
as each voting option differs on head vote, target vote, or source vote.
On expectation, only a few voting options should be available and a supermajority of Validators should pick the same voting option M
after applying the fork-choice rule.
To keep the explanation simple, we assume all validators pick the same message M
, then we end up with a list of public keys and signatures [(P1, σ1), …, (Pn, σn)]
for the same message M
. Once all the votes are cast, the aggregate function allows the aggregator to combine a list of public keys and signatures to be combined into a single public key and signature pair (P, σ)
.
To verify, all the public keys included in the signature can be aggregated together and then they only need to verify the final signature. By checking a single signature — it is in fact checking a collective signature by all Validators in the aggregation.
If you want to learn about the finer details of BLS aggregation, you can find out more here.
Why Subcommittees?
A slot’s committee is a collection of 64 smaller committees. It is a subtle technical detail, but arises due to the previous plans for execution sharding and it may now be leveraged in future plans for data sharding.
Regardless, the subcommittees provide a bandwidth benefit. Aggregators only handle attestations for Validators within their subcommittee. If we assume:
Network has 500k Validators,
A slot’s committee has 15k Validators (500k/32).
Then a subcommittee has approximately ~240 Validators (15k/64 committees) and an aggregator only needs to handle 240 unique attestations which is about ~40k attestation data prior to aggregating it.
Aggregator’s Private Lottery
The target is to elect ~16 aggregators for every subcommittee who will take the attestations, aggregate them, and pass on the final aggregations to the next slot’s block proposer.
The appointment protocol for aggregators has three goals:
Publicly verifiable. Any external observer can verify that a Validator was elected as an aggregator for the slot.
Self-election. A Validator participates in a private lottery and can independently check whether they were appointed as an aggregator.
Secrecy. A Validator can decide to reveal if they were appointed as an aggregator for a slot.
The appointment of aggregators relies upon a private lottery. They can privately check if they hold a winning ticket and decide to publicly announce it to all other Validators in the subcommittee. The winning ticket represents indisputable evidence of their appointment.
Private lottery. We provide pseudocode for the private lottery and dive into it a bit more detail:
slot; // Data about current slot
sub_committee; // Aggregator's assigned subcommittee.
// Compute lotto ticket
signature = BLS.sign(slot.number);
ticket = hash(signature); // Lotto ticket is hash of digital signature
// Check winnings
TARGET_AGGREGATORS_PER_COMMITTEE = 16;
target = sub_committee.length / TARGET_AGGREGATORS_PER_COMMITTEE;
return ticket %% target == 0 ? TRUE : FALSE;
The lottery ticket is a Validator’s digital signature over the current slot number. A hash of the signature, alongside the size of the subcommittee, is used to randomly decide if it is a winning ticket. Since the ticket is a digital signature and the evaluation criteria is publicly available, then anyone can repeat the same computation to check that digital signature is indeed a winning ticket.
The lottery’s integrity relies on a special property of BLS signatures. Given a message and the signing key, it will always produce the same digital signature. The deterministic nature of BLS signatures prevents a Validator from forging a winning ticket. The downside of a probabilistic private lottery for electing aggregators is the potential for an aggregator not to be elected in a single slot.
As a side note, there is no guarantee a subcommittee will have a self-elected aggregator. This should happen to a single subcommittee every ~8 weeks and the the attestations will not be passed to the next slot’s block proposer.
Aggregating Attestations
On aggregator is responsible for collecting the subcommittee’s attestations, aggregating the attestations and passing it onto the next slot’s block proposer.
There are some restrictions an aggregator must take into account:
Subcommittee only. Only attestations from other Validators in the same subcommittee can be aggregated.
Aggregation limit. Up to 2,048 attestations can be aggregated as this is the maximum size of a subcommittee.
Same attestation. Only attestations for the same head, target and source vote can be aggregated and it must reflect the aggregator’s vote.
A little implementation specific, but the aggregator updates a bitmap called aggregation_bits
to indicate which committee member’s signature is included in the aggregation.
The aggregator includes the final aggregated signature, public key and the bitmap in a SignedAggregateAndProof
message. It is signed and passed on after the slot’s 8 second mark to ensure the next slot’s block proposer has sufficient time to receive and process it.
Note, the additional signature to authorise the aggregation is only used by the peer to peer network for propagation purposes and it is not recorded in a beacon block.
Attestation inclusion in a beacon block
The next slot’s block proposer collects aggregations for inclusion in their beacon block. It is the primary source of revenue for a block proposer as they collect 1/8th of the attestation reward and the final reward depends on timeliness of including the attestation.
A block proposer must check the aggregation of attestations before including it:
Get public keys. Fetch corresponding public key for each Validator in the subcommittee based on the flipped bits in
aggregation_bits
.Check aggregation public key. Combine all fetched public keys and compare it with the attested aggregated public key. public keys.
Verify signature. Check the digital signature corresponds to the aggregated public key and the attestation.
Put simply, the block proposer only needs to independently compute the aggregated public key, check its correctness, and verify a single signature. If it passes, it can include the aggregated attestations in their beacon block.
The primary rule a block proposer must follow is that all attestations have the same source vote as the justified block for this blockchain fork. If the source vote is different, then it represents a competing blockchain fork which has been sustained for at least the last 32 slots.
A block proposer has flexibility over the aggregation process:
Aggregate disjoint aggregations. If there are two or more disjoint aggregations for the same subcommittee, a block proposer can aggregate them into a single aggregation.
Multiple aggregations for same subcommittee allowed. If there are two or more aggregations that share a subset of the same attestations, then it is not technically possible to aggregate them.
Overlapping attestations allowed. The proof of stake protocol takes care of double-voting. Only the attestation in the first aggregation is counted.
Interestingly, the ability to record overlapping attestations in the same beacon block has led to more attestations being processed than Validator’s in a subcommittee. The repeated process is simply aggregating the same public key in multiple batches, but it should have little performance overhead.
Finally, there is no reward issued for aggregators who perform this role on the network as the reward can simply be stolen by the block proposer. It is simply a voluntary service offered by aggregators to help speed up the propagation process for recent attestations. As such, there is no accreditation in a beacon block for whoever performed the aggregation.